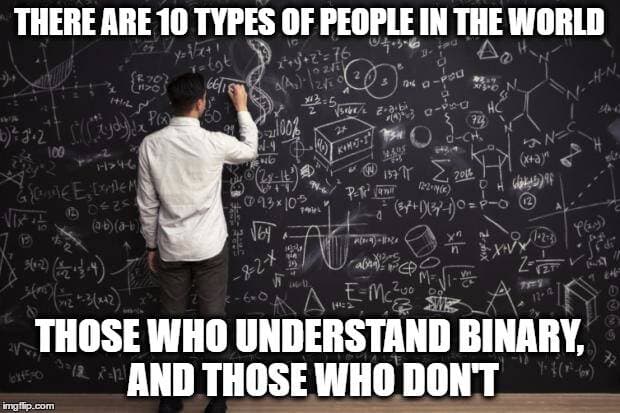
Binary in the Browser: Understanding ArrayBuffer, TypedArray, and DataView
In modern computer science, binary data is the foundation of information storage and transmission. Whether it's files, network protocols, or multimedia data like images and audio, everything is ultimately stored and processed in binary form. To better understand ArrayBuffer
, TypedArray
, and DataView
in JavaScript, we first need to grasp some fundamental binary concepts, including bit, Byte, and Hexadecimal.
A bit is the smallest unit of data in a computer, representing a binary digit that can be either 0
or 1
. For example:
0
represents "off" or "false."1
represents "on" or "true."
Multiple bits can be combined to represent more complex data. For instance, 2 bits can represent 4 states (00
, 01
, 10
, 11
), 3 bits can represent 8 states, and so on.
A Byte is a common unit of data in computers, consisting of 8 bits. One Byte can represent 256 different states (from 00000000
to 11111111
, i.e., 0
to 255
).
- 1 Byte = 8 bits
- 1 KB (Kilobyte) = 1024 Bytes
- 1 MB (Megabyte) = 1024 KB
- 1 GB (Gigabyte) = 1024 MB
Hexadecimal is a concise way to represent binary data. It uses 16 symbols (0-9
and A-F
) to represent the value of a Byte. For example:
- Binary
00000000
= Hexadecimal0x00
= Decimal0
- Binary
11111111
= Hexadecimal0xFF
= Decimal255
- Binary
10101010
= Hexadecimal0xAA
= Decimal170
Hexadecimal is advantageous because it provides a more compact representation of binary data while remaining human-readable.
In computers, binary data is typically stored and read in Bytes. For example, a 16-bit integer (2 Bytes) can be stored as two consecutive Bytes, while a 32-bit floating-point number (4 Bytes) can be stored as four consecutive Bytes.
When storing multi-byte data, computer systems write the data into memory in a specific order, known as Endianness:
- Big-Endian: The high-order byte is stored at the lower address, and the low-order byte is stored at the higher address.
- Little-Endian: The low-order byte is stored at the lower address, and the high-order byte is stored at the higher address.
For example, the 16-bit integer 0x1234
is stored as follows:
- Big-Endian:
0x12
(high-order byte) is stored at the lower address, and0x34
(low-order byte) is stored at the higher address. - Little-Endian:
0x34
(low-order byte) is stored at the lower address, and0x12
(high-order byte) is stored at the higher address.
JavaScript provides tools like ArrayBuffer
, TypedArray
, and DataView
to efficiently handle binary data.
ArrayBuffer
is an object in JavaScript used to represent a contiguous block of memory. It stores raw binary data but does not directly manipulate it. Instead, ArrayBuffer
acts as a "container," and we need other views (like TypedArray
or DataView
) to access and manipulate the data.
const buffer = new ArrayBuffer(16); // Create a 16-byte buffer
TypedArray
is a set of array views that allow us to access ArrayBuffer
data using specific data types (e.g., Int8
, Uint16
, Float32
, etc.). Common TypedArray
types include:
Int8Array
: 8-bit signed integerUint8Array
: 8-bit unsigned integerInt16Array
: 16-bit signed integerUint16Array
: 16-bit unsigned integerFloat32Array
: 32-bit floating-point numberFloat64Array
: 64-bit floating-point number
const buffer = new ArrayBuffer(16);
const int16Array = new Int16Array(buffer); // Access buffer as 16-bit signed integers
int16Array[0] = 0x1234; // Write data
console.log(int16Array[0].toString(16)); // Read data, outputs "1234"
DataView
is another way to access ArrayBuffer
, providing flexible methods to read and write different types of data.
Unlike TypedArray
, DataView
allows us to read or write various data types in the same ArrayBuffer
without creating multiple views.
const buffer = new ArrayBuffer(16);
const view = new DataView(buffer);
view.setInt16(0, 0x1234, true); // Write a 16-bit signed integer at byte 0 (Little-Endian)
view.setFloat32(4, 3.14, true); // Write a 32-bit floating-point number at byte 4 (Little-Endian)
console.log(view.getInt16(0, true).toString(16)); // Read data at byte 0, outputs "1234"
console.log(view.getFloat32(4, true)); // Read data at byte 4, outputs 3.14
Now, let's demonstrate the practical use of ArrayBuffer
, TypedArray
, and DataView
by writing a function to detect the system's endianness.
The function writes a known value to an ArrayBuffer
and then reads its bytes to determine the endianness.
function detectEndianness() {
// Create a 2-byte ArrayBuffer
const buffer = new ArrayBuffer(2);
const view = new DataView(buffer);
// Write a 16-bit integer 0x1234
view.setUint16(0, 0x1234, true); // Use Little-Endian
// Read the first byte
const firstByte = view.getUint8(0);
// Determine endianness
if (firstByte === 0x34) {
return 'Little-Endian'; // Low-order byte at lower address
} else if (firstByte === 0x12) {
return 'Big-Endian'; // High-order byte at lower address
} else {
return 'Unknown';
}
}
console.log(detectEndianness()); // Outputs the system's endianness
- Write Data: We use
DataView.setUint16
to write0x1234
into theArrayBuffer
, specifying Little-Endian (true
). If the system is Little-Endian,0x34
will be stored at the lower address; if it's Big-Endian,0x12
will be stored at the lower address. - Read Data: We use
DataView.getUint8
to read the first byte. If the value is0x34
, the system is Little-Endian; if it's0x12
, the system is Big-Endian. - Return Result: Based on the read value, the function returns the system's endianness.
Binary data is the foundation of computer science, and JavaScript's ArrayBuffer
, TypedArray
, and DataView
provide powerful tools for handling binary data.
By mastering binary basics, we can better understand how these tools work and apply them effectively in real-world development.